React, the powerful JS library from Facebook 🚀
React is establishing itself as a key player in the front-end. Whether you are a seasoned developer or a beginner, it is crucial to understand the strengths and limitations of this JavaScript library, while exploring its essential features, including hooks
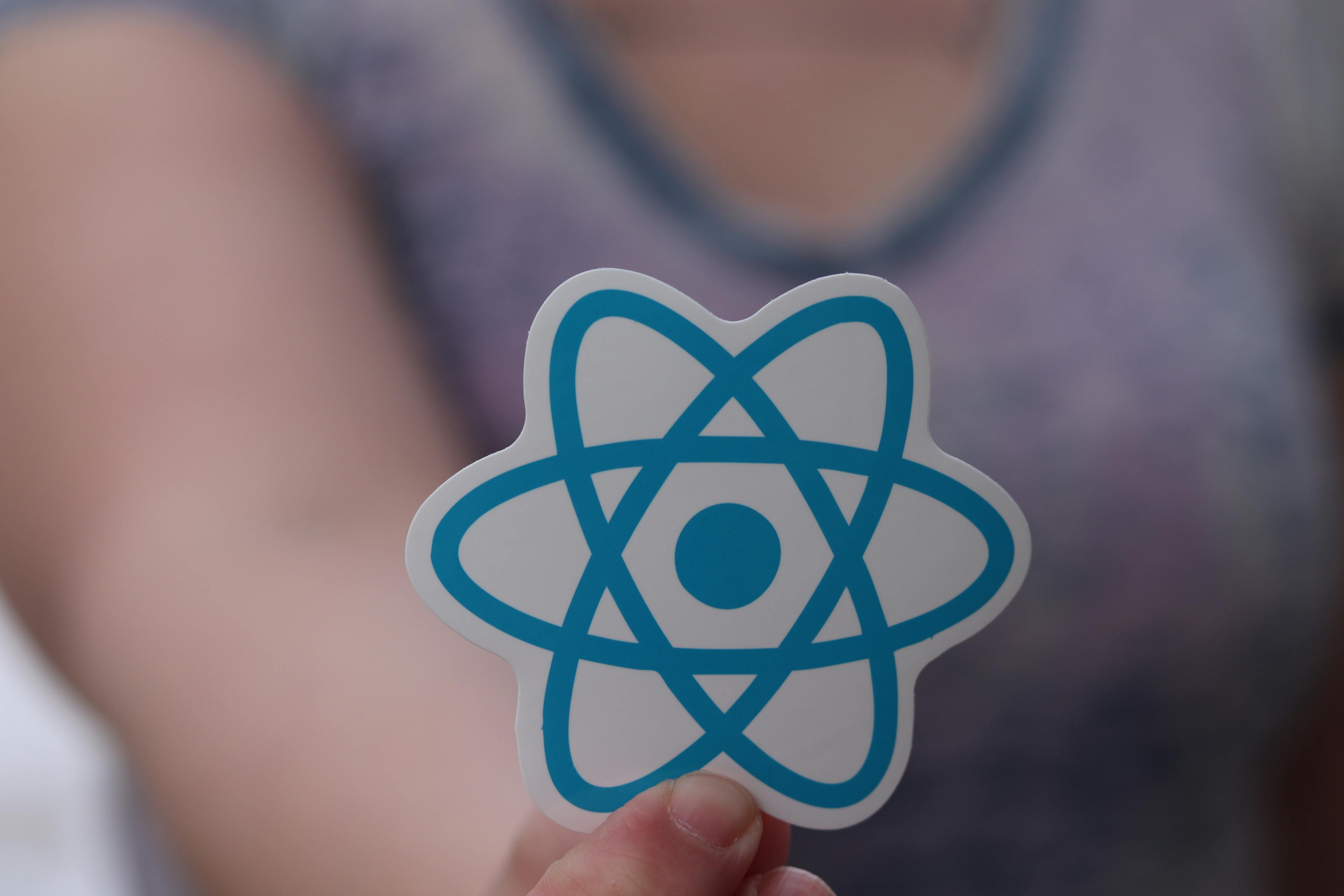
React, the powerful JS library from Facebook 🚀
The fundamentals of React
React, created by Facebook, is an open-source library that makes it easy to create interactive user interfaces. It is based on a model of reusable components. Instead of designing entire web pages, developers build independent components, from the smallest unit (buttons, forms) to more complex elements (control panels, widgets).
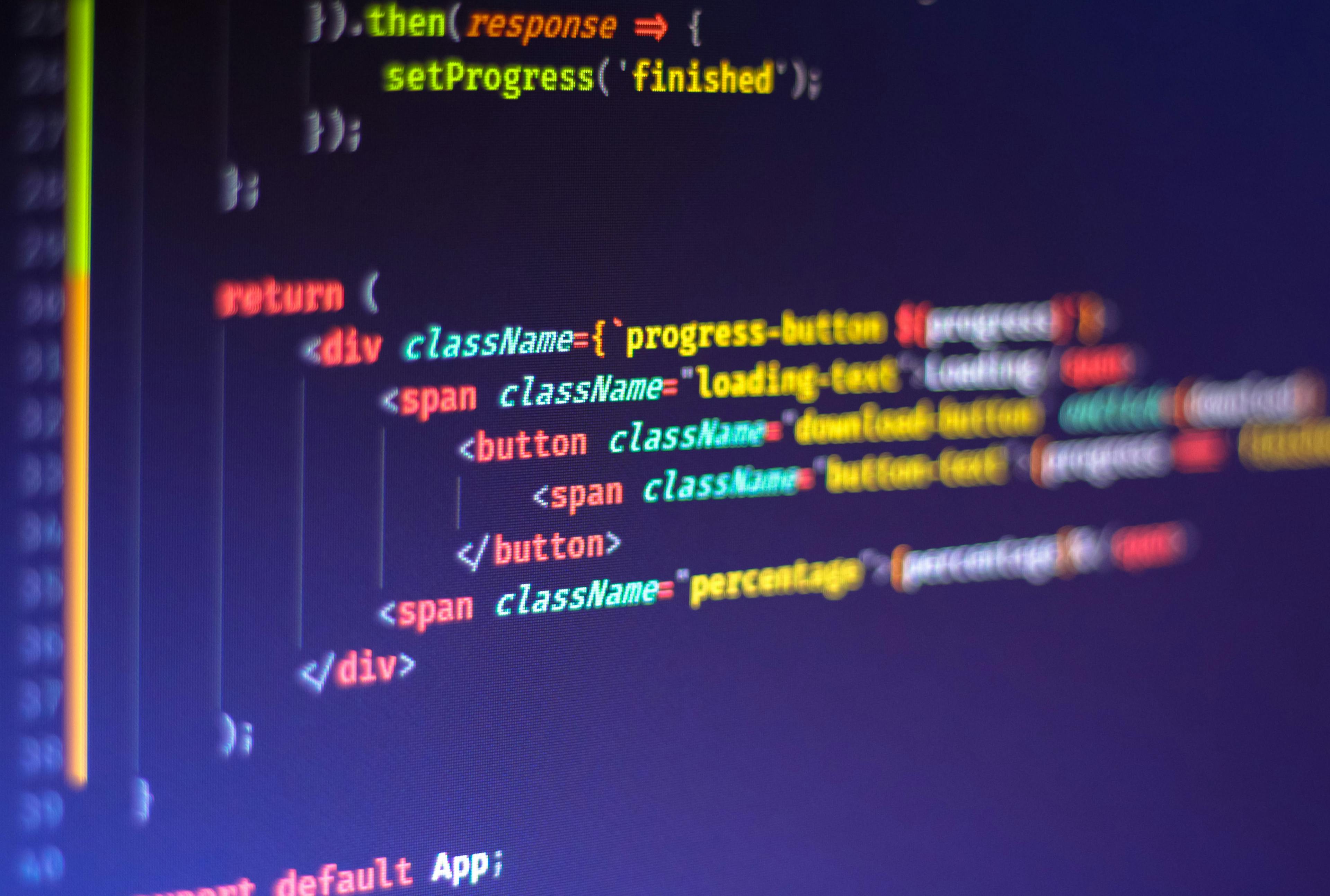
Virtual DOM
One of the most prominent features of React is the Virtual DOM. Instead of directly manipulating the browser's Document Object Model (DOM), React creates a virtual representation of the DOM. When a change is made, React compares the previous Virtual DOM with the new version and updates the real DOM only when necessary. This helps optimize performance by reducing the number of DOM updates.
Some numbers
Among the 3 most popular libraries/frameworks in JavaScript (Angular, React and Vue), React is by far the most downloaded. According to npm-stat.com in December 2023 here are the figures:
- Angular: 441,171 downloads per week.
- React: 21,286,476 downloads per week.
- View: 4,364,740 downloads per week.
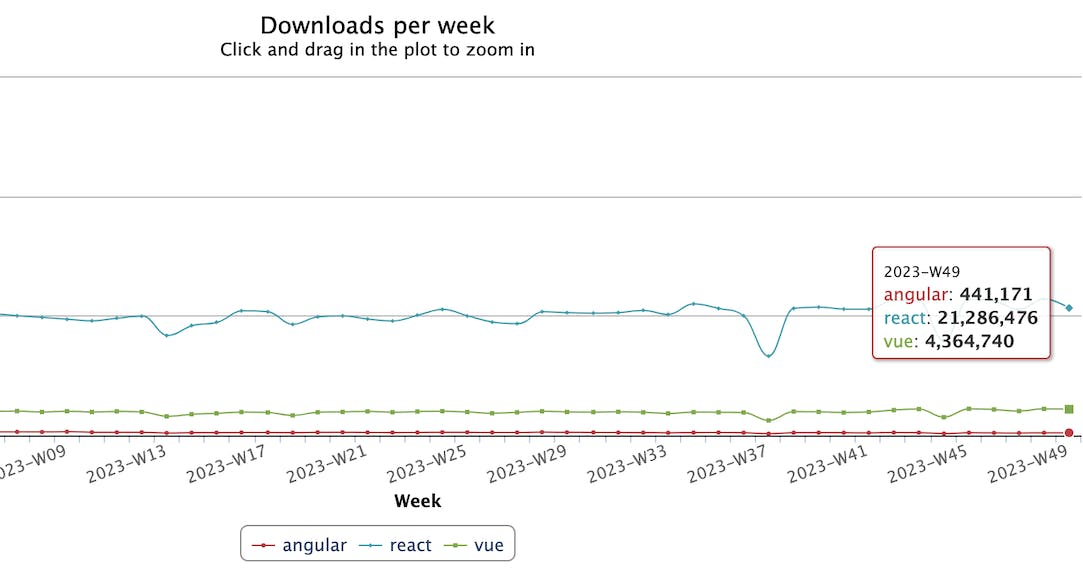
The advantages of React
- 1. Reusable components
- React components are self-contained and reusable. This modularity facilitates maintenance and reuse of code. Components can be used throughout the project, promoting consistency in the user interface.
- 2. An active community
- The React community is large and active. It offers a rich ecosystem of third-party libraries, tools and learning resources. Whether you need to manage state, add animations, or create complex user interfaces, you'll likely find a pre-existing solution.
- 3. SEO-friendly
- React web applications can be optimized for SEO using libraries like Next.js. Server-side rendering allows search engines to easily index content, thereby improving app visibility.
The disadvantages of React
- 1. Learning curve
- React can seem complex for beginners. Creating components, managing state with the concept of "state", and using JSX take time to master. However, this learning curve is an essential step to fully exploit the benefits of React.
- 2. Complexity of major projects
- Large-scale React applications can get complex. State management, network requests, routing, and data manipulation can result in code that is difficult to maintain. A solid structure and development discipline are essential.
- 3. Heavy JavaScript
- React, using JavaScript, can result in heavier applications in terms of file size and loading time. Code fragmentation should be avoided by using techniques such as code splitting to improve performance.
- 4. Multiple choices
- The React ecosystem offers many options for state management, routing, testing, and more. This abundance of choice can make selecting the best solutions difficult, which can lead to code fragmentation.
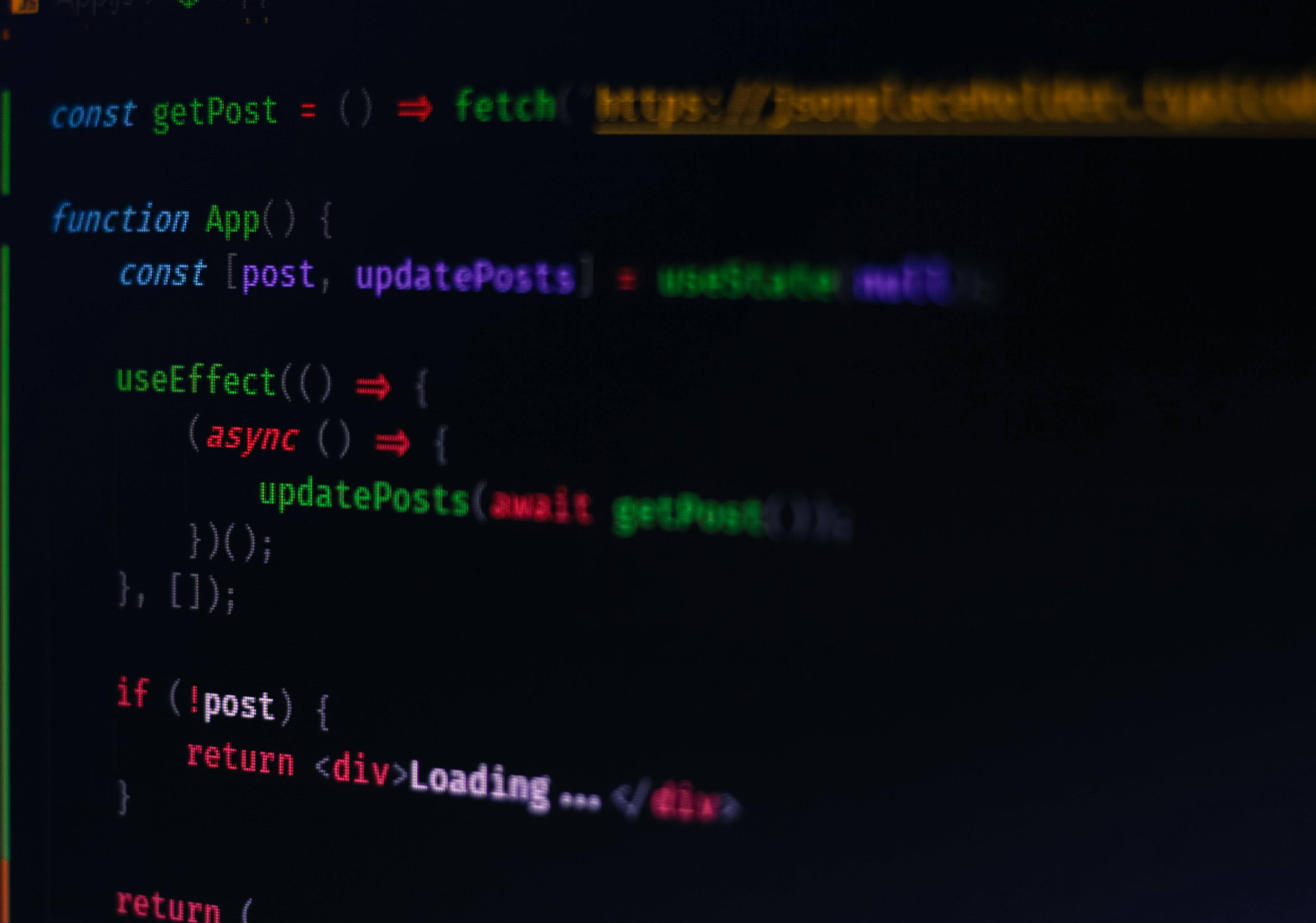
Exploring React Hooks
Now, let's dive into one of the most powerful features of React: hooks. Hooks are functions that allow functional components to manage state and effects, opening the way to new development possibilities. Here are some of the most commonly used hooks:
1. useState
The useState hook allows you to manage local state in a functional component. It allows you to declare state variables and update them, which triggers the component to render when they change.
import { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
export default Counter;
2. useEffect
The useEffect hook is used to perform side effects in a component. It can be used to handle network calls, DOM updates, and more, in response to state changes.
import { useState, useEffect } from 'react';
const DataFetcher = () => {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data d'une API
fetch('https://api.example.com/data')
.then(response => response.json())
.then(result => setData(result))
.catch(error => console.error('Error fetching data:', error));
}, []); // An empty dependency array means this effect runs once after initial rendering
return (
<div>
<h2>Data:</h2>
{data ? <p>{data}</p> : <p>Loading...</p>}
</div>
);
}
export default DataFetcher;
3. useRef
The useRef hook allows you to create references to DOM elements or persistent values, without triggering new renderings of the component.
import { useRef, useEffect } from 'react';
const FocusInput = () => {
const inputRef = useRef(null);
useEffect(() => {
// Focus on the input element when mounting the component
inputRef.current.focus();
}, []);
return <input ref={inputRef} type="text" placeholder="Type here" />;
}
export default FocusInput;
4. useContext
The useContext hook provides access to a shared context in the component tree. This makes it easier to manage shared data between components, avoiding the need to pass properties from component to component.
5. useReducer
The useReducer hook is useful for handling more complex states by combining useState and useEffect. It allows you to centralize the management of the state of a component and perform more controlled updates.
Conclusion
Choosing React for a web development project is similar to planning a trip. You'll explore a world of benefits, such as reusable components, Virtual DOM, an active community and SEO support. However, it's essential to consider the learning curve, potential complexity, JavaScript load, and abundance of choices before you take the plunge.
React hooks are a great asset for simplifying the management of state and effects in functional components. They open up a universe of possibilities for creating dynamic and interactive web applications. I invite you to discover the projects I have carried out with React and its great framework Next.js!
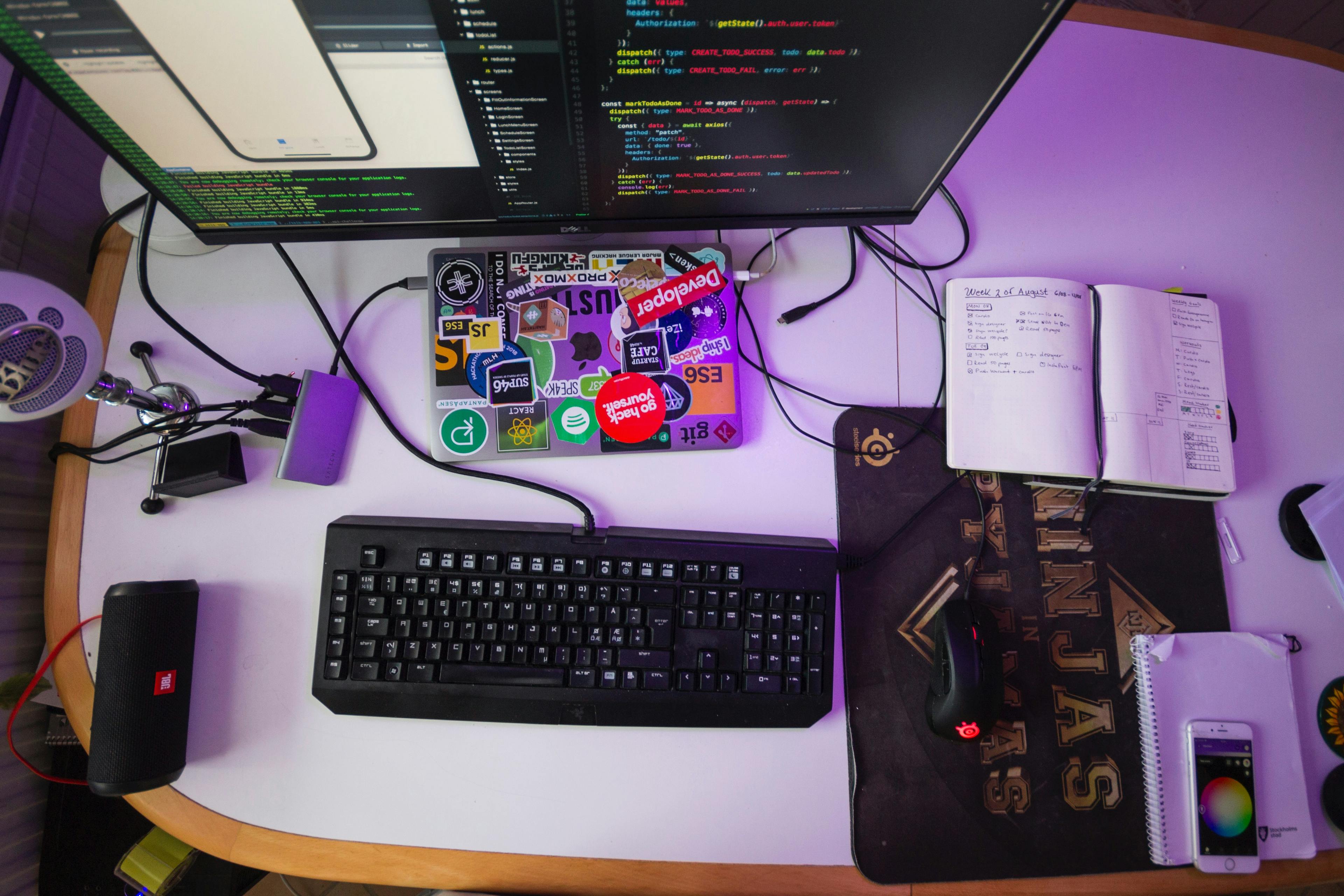
Commentaires
Connectez-vous pour ajouter un commentaire
Se connecter avec Google